|
 |
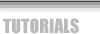 |
 |
|
JSON Your AJAX
By Gal Ratner
One of the most requested features of web pages today is partial refreshes.
The ability to update an element on the page from the server without refreshing the page is now almost the norm on data driven websites.
Users love it. It cuts down on loading time and network traffic. The idea and implementation is not new.
It is based on Microsoft's XMLHTTPRequest object that was released with Internet Explorer 5 in 2003.
Since then all of the major browsers have implemented this feature. This is most commonly known as Asynchronous JavaScript and XML or AJAX for short.
The data coming from the server will be returned as a string. This can be an XML formatted string if the URL invoked is a web service.
The code will look like this:
var xmlHttp;
if(window.XMLHttpRequest) // Are we on Firefox, Safari or Opera?
xmlHttp=new XMLHttpRequest();
else // IE
xmlHttp=new ActiveXObject("Msxml2.XMLHTTP");
Let's request a page and display the result in an alert box:
xmlhttp.Open("GET", "yourpage.aspx", false); // The method GET|POST,
// your URL to invoke and false for a synchronously read. The script will wait for a result.
xmlhttp.send();
alert(xmlhttp.responseText);
The xmlHttp object is event driven and can notify us asynchronously as things happen.
We will have to register a listener to its state and act in order to send and receive data.
The event we are going to trap is onreadystatechange.
xmlHttp.onreadystatechange=function()
{
if(xmlHttp.readyState==0) // The request is not initialized
{
}
else if(xmlHttp.readyState==1) // The request has been set up
{
}
else if(xmlHttp.readyState==2) // The request has been sent
{
}
else if(xmlHttp.readyState==3) // The request is in process
{
// Desplay a loading bar
}
else if(xmlHttp.readyState==4) // The request is complete
{
// Get the data here
if (xmlhttp.status==200) // The page is there
{
// Do something with the data
alert(xmlhttp.responseText);
}
}
}
Now let's open a URL and read from it
xmlHttp.Open("GET","yourpage.aspx",true);
xmlHttp.send(null); //We are not sending anything here, just reading data.
XMLHTTP can also post data as a binary stream:
xmlHttp.Open("POST","yourpage.aspx",true);
xmlhttp.setRequestHeader("Content-Type", "application/x-www-form-urlencoded");
xmlHttp.send(param1=value1¶m2=value2);
This presented two major problems:
Security - The data coming from the server will have to be on the same domain. XMLHTTPRequest will not cross domains.
Formatting - The data is returned as text. This means you will have to write your own Javascript text parser to read it and extract the data in order to display it.
Here comes JSON
JSON - JavaScript Object Notation is a way to arrange your returned data as a Javascript object.
This is done on the server so all you have to do it load the result and start using the data as if you would on any other data object
The result will look like this:
{
"Field1": "Value1",
" Field2": " Value2",
" Field3": {
" SubField3": " Value3",
" SubField3a": "Value4"
},
}
All we have to do now is to Eval it into an object:
if (xmlhttp.status==200) // The page is there
{
var JSONObject = eval("(" + xmlHttp.responseText + ")");
}
We are now free to use its fields:
JSONObject.Field1 contains "Value1"
document.getElementById(your element to set with the new text).text = JSONObject.Field1;
Getting across domains:
Just add the result as a script
<script language=JavaScript src="JSONEnabledPage.aspx"></script>
That was easy!
Now let's do it using ASP.NET AJAX Framework from Microsoft.
The framework can be downloaded for free here and you need Visual Studio or Visual Web Developer available as a free download here in order to use the Framework.
Let's start with backend web service:
Create a new web service and add the imports
using System.Web.Services;
using System.Web.Services.Protocols;
Now add the tags
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.Web.Script.Services.ScriptService()]
Above the class declaration and add the following method:
[WebMethod]
public List GetListOfWords()
{
List words = new List();
words.add("Hello");
words.add("This");
words.add("Is");
words.add("A");
words.add("JSON");
words.add("Test");
return words;
}
On your ASPX page add to the to the script manager the location of your service:
<asp:ScriptManager ID="ScriptManager1" runat="server">
<Services>
<asp:ServiceReference Path="YourWebService.asmx" />
</Services>
</asp:ScriptManager>
Invoke the remote method
ret = YourClass. GetListOfWords(OnSendComplete, OnSendTimeOut, OnSendError);
and wait for the response
function OnSendComplete(args) {
for(var i=0;i<args.length;i++){
alert(args);
}
}
If you are sending an object or an array of objects, use args.YourProperty or args[i].YourProperty to access any values inside.
That's it! Quick and easy. Make parcial page refreshes a part of your next web application Your users will love it!
|
|
|
 |
|
 |
About Us | Contact Us | Standards of Business Conduct | Employment
© InvertedSoftware.com All rights reserved
|